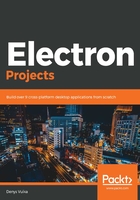
Sending messages to the renderer process
Now, we can send messages from the main process back to the renderer. According to our initial scenario, we are going to handle application menu clicks and let the renderer process know about user interactions.
To send messages to the renderer process, we need to know what window we should address. Electron supports multiple windows with different content, and our code needs to know or figure out which window contains the editor component. For the sake of simplicity, let's access the focused window object since we have only a single-window application right now:
- Import the BrowserWindow object from the Electron framework:
const { BrowserWindow } = require('electron');
The format of the call is as follows:
const window = BrowserWindow.getFocusedWindow();
window.webContents.send('<channel>', args);
At this point, we have communication handlers from both areas, that is, the browser and Node.js. It is time to wire everything with a menu item.
- Update your menu.js file and provide a Toggle Bold entry that sends a toggle-bold message using our newly introduced editor-event channel. Refer to the following code for implementation details:
const template = [
{
label: 'Format',
submenu: [
{
label: 'Toggle Bold',
click() {
const window = BrowserWindow.getFocusedWindow();
window.webContents.send(
'editor-event',
'toggle-bold'
);
}
}
]
}
];
Let's check whether the messaging process works as expected.
- Run the application with the npm start command, or restart it, and toggle the Developer Tools.
- Note that you also have the Format menu, which contains the Toggle bold subitem. Click it and see what happens in the browser console output in the Developer Tools:

- The Terminal output should contain the following text:
> DEBUG=true electron .
Received reply from web page: Page Loaded
Received reply from web page: Received toggle-bold
This is a great result! As soon as we click on the application menu button, the main process finds the focused window and sends the toggle-bold message. The renderer process handles the message in Javascript and posts it to the browser console. After that, it replies to the message, and the main process receives and outputs the response in the Terminal window.