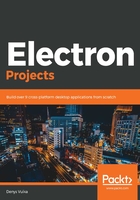
Sending confirmation messages to the main process
You can also send messages back to the main process with the send function:
ipcRenderer.send('<channel-name>', arg);
As an exercise, let's send a confirmation back to the main process. Electron provides convenient access to the sender of the message via the event argument. This allows us to have generic message handlers wired with multiple channels.
The Node.js part of the application is going to listen to the editor-reply channel to receive feedback from the web page.
- Update the code of the index.html page to reflect the following example:
<script>
const { ipcRenderer } = require('electron');
ipcRenderer.on('editor-event', (event, arg) => {
console.log(arg);
// send message back to main process
event.sender.send('editor-reply', `Received ${arg}`);
});
</script>
- At the renderer side, we need to create a reply handler. First, we need to import the ipcMain project from the Electron framework. Update the menu.js file and add the following import to the top of the file:
const { ipcMain } = require('electron');
- Next, write the handler, similar to what we did for the web page scripts:
ipcMain.on('editor-reply', (event, arg) => {
console.log(`Received reply from web page: ${arg}`);
});
To keep things simple and understandable, we also put the content of the message in the output.
Now, it's time to see the messages go from the renderer to the main process.
- For testing purposes, append the following code to the bottom of the script in the index.html page:
ipcRenderer.send('editor-reply', 'Page Loaded');
- The whole script block should look as follows:
<script>
var editor = new SimpleMDE({
element: document.getElementById('editor')
});
const { ipcRenderer } = require('electron');
ipcRenderer.on('editor-event', (event, arg) => {
console.log(arg);
event.sender.send('editor-reply', `Received ${arg}`);
});
ipcRenderer.send('editor-reply', 'Page Loaded');
</script>
As you can see, as soon as the page is rendered to the users, the script sends the Page Loaded message to the main process while utilizing the editor-reply channel. We enabled logging to the console for all reply messages once you run your application with the npm start script, the command's output should contain the following text:
> DEBUG=true electron .
Received reply from web page: hello world
This message means that your first messaging channel works from the renderer process to the main one.