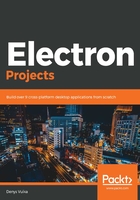
Defining menu item roles
The Electron framework supports a set of standard actions that you can associate with menu items. Instead of providing a label text, click handlers, and other settings, you can pick one of the role presets, and the Electron shell will handle it on the fly. Using menu presets saves a lot of time and effort as you don't need to type a lot of code to replicate standard and system entries.
Let's learn how to run Chrome's Developer Tools from our custom menu, without writing a single line of code in JavaScript:
- Switch back to the menu template in the menu.js file and insert the following block to create a new Debugging menu:
const template = [
{
role: 'help',
submenu: [
{
label: 'About Editor Component',
click() {
shell.openExternal('https://simplemde.com/');
}
}
]
},
{
label: 'Debugging',
submenu: [
{
role: 'toggleDevTools'
}
]
}
];
Note how we set only a single attribute, that is, role, to the value of toggleDevTools in the submenu array. toggleDevTools is one of the numerous predefined roles that the Electron framework supports. With a single role reference, your application usually gets a label, keyboard shortcut, and a click handler. In some cases, you may get even a complex menu structure with child items, such as when you use a Help role.
- Run the application to see the toggleDevTools role in action:
npm start
Note that you now have two custom top-level menus. One of those is Debugging, which contains the Toggle Developer Tools menu item. Once you click it, you should get the standard Chrome Developer Tools on your screen:

- Changing the title of the predefined role item is easy. Just add the label attribute, as shown in the following code:
{
label: 'Debugging',
submenu: [
{
label: 'Dev Tools',
role: 'toggleDevTools'
}
]
}
- Now, if you run the application once again, the title of the menu item will be Dev Tools, but the behavior is still the same—it opens Chrome's Developer Tools when it's clicked.
A typical application may contain lots of menu items. In the next section, we are going to learn how to gather actions into groups and use menu separators.